This is how you use SVG in your React UI
How to turn your SVG into React components in only 2 clicks
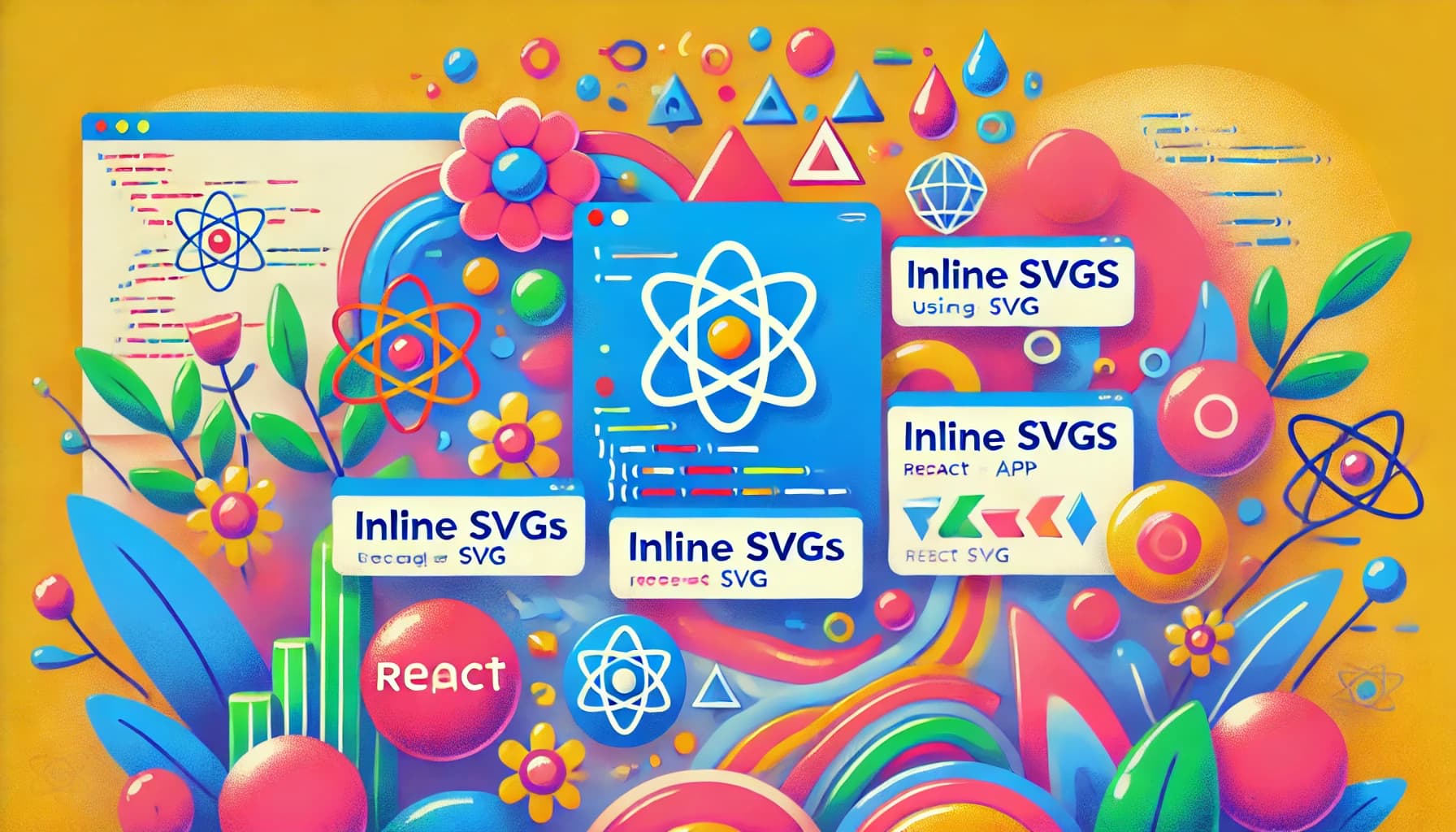
Introduction
In modern web development, Scalable Vector Graphics (SVG) have become an essential part of building responsive, high-quality interfaces. SVG provide several advantages over traditional image formats, including scalability without loss of quality, smaller file sizes, and the ability to manipulate through CSS and JavaScript. In this article, we’ll explore how to effectively use SVG in a React application.
Why Use SVG?
- Scalability: SVG can scale to any size without losing quality, making them perfect for responsive designs.
- Performance: Typically, SVG files are smaller than raster images like PNG or JPEG, leading to faster load times.
- Styling and Interactivity: SVG can be styled with CSS and made interactive with JavaScript, allowing for dynamic and engaging user interfaces.
How to Use Inline SVG in React
There are various ways to include SVG in your React app, but using inline SVG provides maximum flexibility. Here’s a step-by-step guide:
Step 1: Creating a Simple Inline SVG Component
Start by creating a functional component that includes your SVG code directly within the JSX. Here's a basic example with a star shape:
import React from 'react';
const Star = () => (
<svg
width="100"
height="100"
viewBox="0 0 24 24"
fill="gold"
xmlns="http://www.w3.org/2000/svg"
>
<path d="M12 2L14.09 8.26L20.97 8.27L15.36 12.14L17.45 18.4L12 14.53L6.55 18.4L8.64 12.14L3.03 8.27L9.91 8.26L12 2Z" />
</svg>
);
export default Star;
Step 2: Adding Dynamic Properties
To make your SVG component more versatile, you can add props for dynamic properties like color and size:
import React from 'react';
const Star = ({ size = 100, color = 'gold' }) => (
<svg
width={size}
height={size}
viewBox="0 0 24 24"
fill={color}
xmlns="http://www.w3.org/2000/svg"
>
<path d="M12 2L14.09 8.26L20.97 8.27L15.36 12.14L17.45 18.4L12 14.53L6.55 18.4L8.64 12.14L3.03 8.27L9.91 8.26L12 2Z" />
</svg>
);
export default Star;
Now, you can customize the Star component's color and size when you use it:
<Star size={150} color="blue" />
✨ Hold on, there is a better way!
If you don't want to go through the manual labor of creating components out of your SVG, you can simply use ReactSVG and we'll create the React components for you.
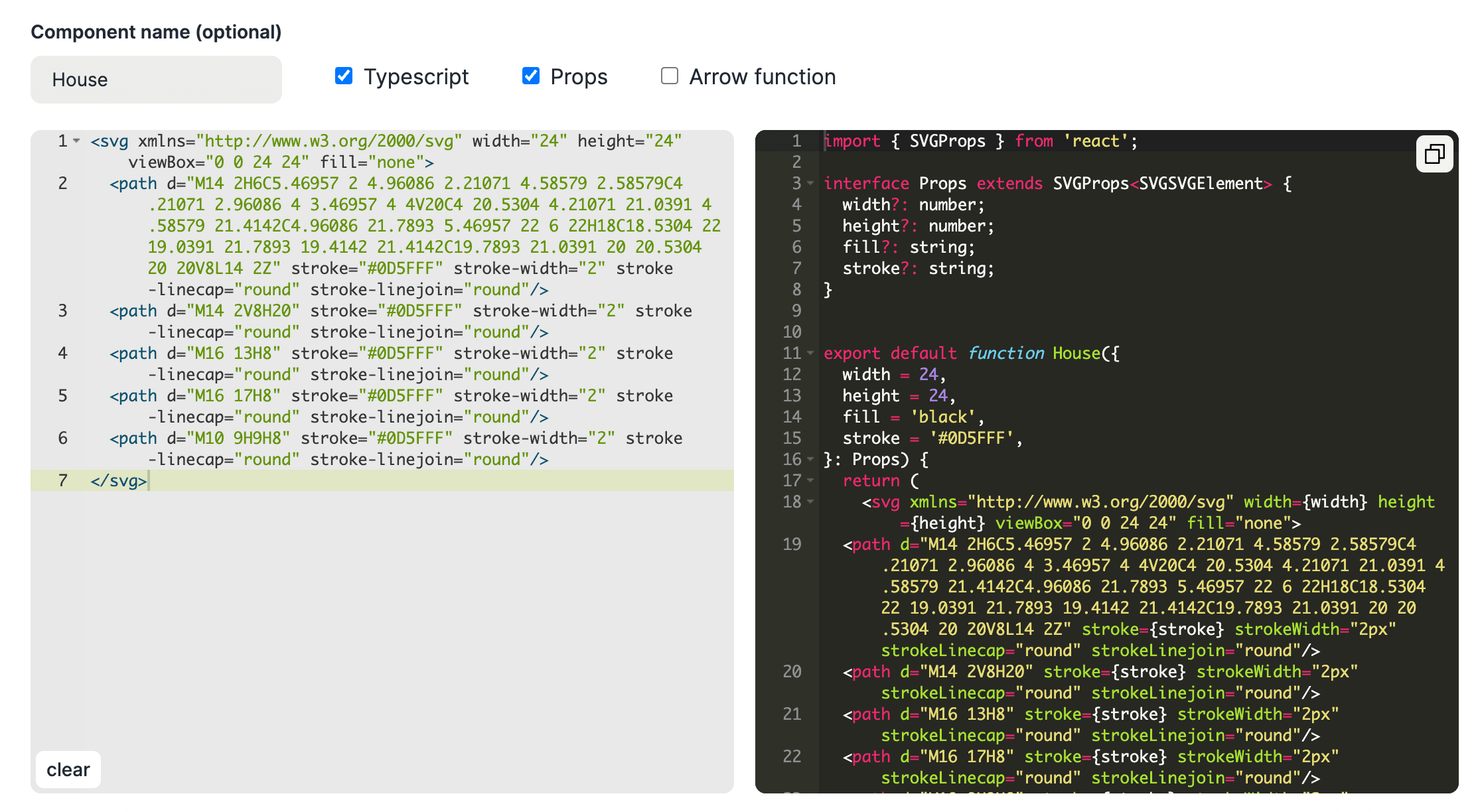
Conclusion
Using inline SVGs in your React app is a game-changer for creating scalable, high-quality graphics that are both interactive and dynamic. Use ReactSVG to easily integrate SVGs into your components and take full advantage of what they offer. Play around with different properties, styles, and animations to make your user interfaces more engaging and visually appealing. Give it a try and see how SVGs can elevate your React projects!